Model.addVars()
addVars ( *indices, lb=0.0, ub=GRB.INFINITY, obj=0.0, vtype=GRB.CONTINUOUS, name="" )
Add multiple decision variables to a model.
Returns a Gurobi tupledict
object that contains the newly created variables. The keys for the
tupledict
are derived from the indices
argument(s). The
arguments for this method can take several different forms, which will
be described now.
The first arguments provide the indices that will be used as keys to
access the variables in the returned tupledict
. In its
simplest version, you would specify one or more integer values, and
this method would create the equivalent of a multi-dimensional array
of variables. For example, x = model.addVars(2, 3)
would
create six variables, accessed as x[0,0]
, x[0,1]
,
x[0,2]
, x[1,0]
, x[1,1]
, and x[1,2]
.
In a more complex version, you can specify arbitrary lists of
immutable objects, and this method will create variables for each
member of the cross product of these lists. For example,
x = model.addVars([3, 7], ['a', 'b', 'c'])
would create
six variables, accessed as x[3,'a']
, x[7,'c']
, etc.
You can also provide your own list of tuples as indices. For example,
x = model.addVars([(3,'a'), (3,'b'), (7,'b'), (7,'c')])
would
be accessed in the same way as the previous example (x[3,'a']
,
x[7,'c']
, etc.), except that not all combinations will be
present. This is typically how sparse indexing is handled.
Note that while the indices can be provided as multiple lists of
objects, or as a list of tuples, the member values for a specific
index must always be scalars (int
, float
, string
,
...). For example, x = model.addVars([(1, 3), 7], ['a'])
is
not allowed, since the first argument for the first member would be
(1, 3)
. Similarly,
x = model.addVars([((1, 3),'a'), (7,'a')])
is also not allowed.
The named arguments (lb
, obj
, etc.) can take several
forms. If you provide a scalar value (or use the default), then every
variable will use that value. Thus, for example, lb=1.0
will
give every created variable a lower bound of 1.0. Note that a scalar
value for the name argument has a special meaning, which will be
discussed separately.
You can also provide a Python dict
as the argument. In that
case, the value for each variable will be pulled from the dict, using
the indices argument to build the keys. For example, if the variables
created by this method are indexed as x[i,j]
, then the
dict
provided for the argument should have an entry for each
possible (i,j)
value.
Finally, if your indices
argument is a single list, you can
provide a Python list
of the same length for the named
arguments. For each variable, it will pull the value from
the corresponding position in the list.
As noted earlier, the name
argument is special. If you provide
a scalar argument for the name, that argument will be transformed to
have a subscript that corresponds to the index of the associated
variable. For example, if you do
x = model.addVars(2,3,name="x")
, the variables will get names
x[0,0]
, x[0,1]
, etc.
Arguments:
indices: Indices for accessing the new variables.
lb (optional): Lower bound(s) for new variables.
ub (optional): Upper bound(s) for new variables.
obj (optional): Objective coefficient(s) for new variables.
vtype (optional): Variable type(s) for new variables.
name (optional): Names for new variables. The given name will be subscripted by the index of the generator expression, so if the index is an integer, c would become c[0], c[1], etc. Note that the generated names will be stored as ASCII strings, so you should avoid using names that contain non-ASCII characters. In addition, names that contain spaces are strongly discouraged, because they can't be written to LP format files.
Return value:
New tupledict object that contains the new variables as values, using the provided indices as keys.
Example usage:
# 3-D array of binary variables x = model.addVars(3, 4, 5, vtype=GRB.BINARY) # variables index by tuplelist l = tuplelist([(1, 2), (1, 3), (2, 3)]) y = model.addVars(l, ub=[1, 2, 3])
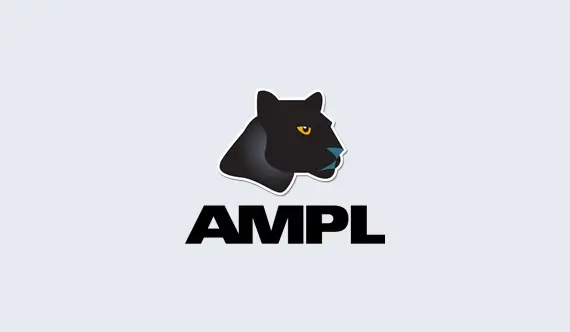
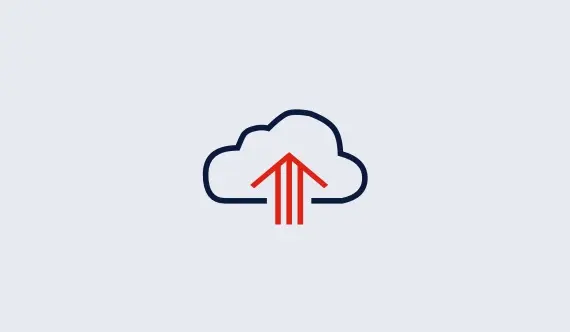
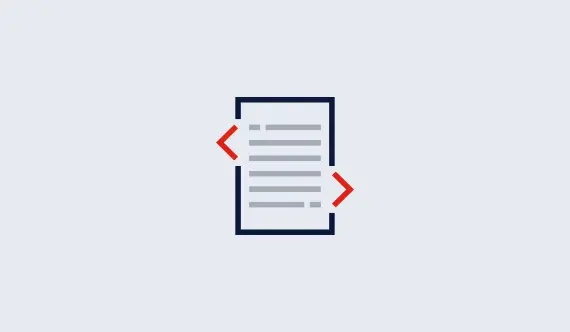
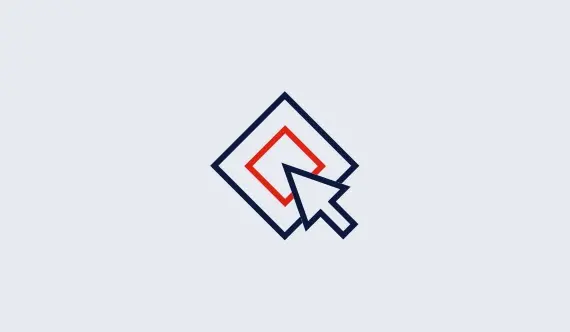
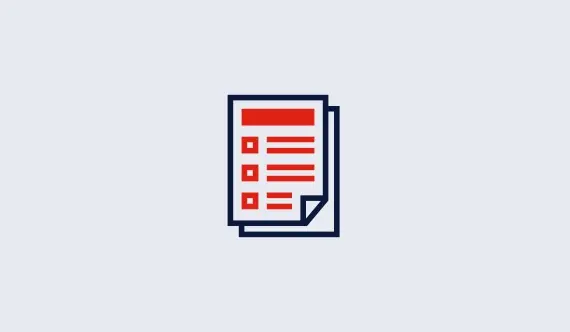
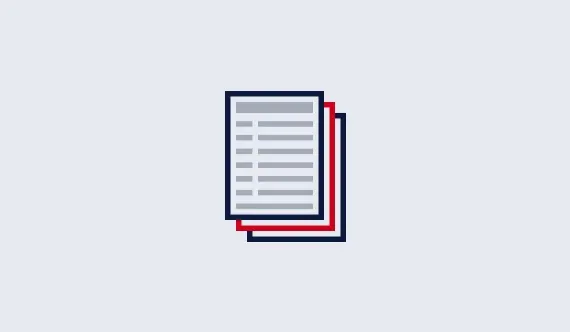
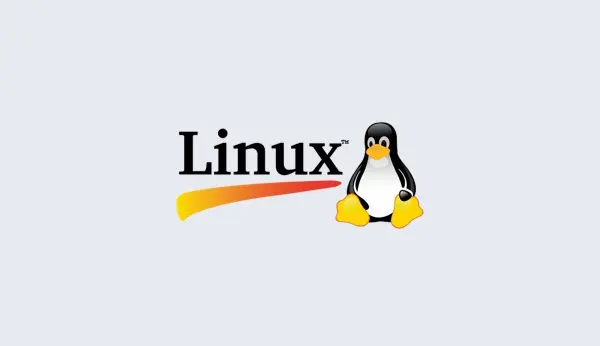
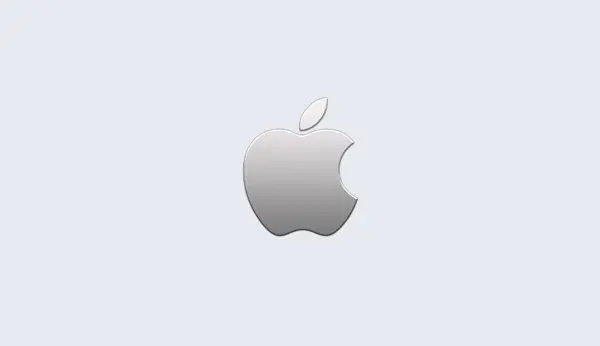
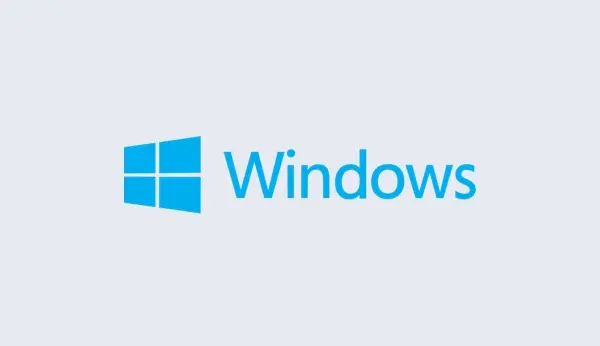