Customization through callbacks
Another type of customization we'd like to touch on briefly can be achieved through Gurobi callbacks. Callbacks allow you to track the progress of the optimization process. For the sake of our example, let's say you want the MIP optimizer to run for 10 seconds before quitting, but you don't want it to terminate before it finds a feasible solution. The following callback method would implement this condition:
from gurobipy import * def mycallback(model, where): if where == GRB.Callback.MIP: time = model.cbGet(GRB.Callback.RUNTIME) best = model.cbGet(GRB.Callback.MIP_OBJBST) if time > 10 and best < GRB.INFINITY: model.terminate()
Once you import this function (from custom import *
), you
can then say m.optimize(mycallback)
to obtain the desired
termination behavior. Alternatively, you could define your own custom
optimize method that always invokes the callback:
def myopt(model): model.optimize(mycallback)This would allow you to say
myopt(m)
.
You can pass arbitrary data into your callback through the model
object. For example, if you set m._mydata = 1
before calling
optimize(), you can query m._mydata
inside your
callback function. Note that the names of user data fields must begin
with an underscore.
This callback example is included in
<installdir>/examples/python/custom.py
.
Type from custom import *
to import the callback and the
myopt() function.
You can type help(GRB.Callback)
for more information on
callbacks. You can also refer to the Callback
class
documentation in the Gurobi Reference
Manual.
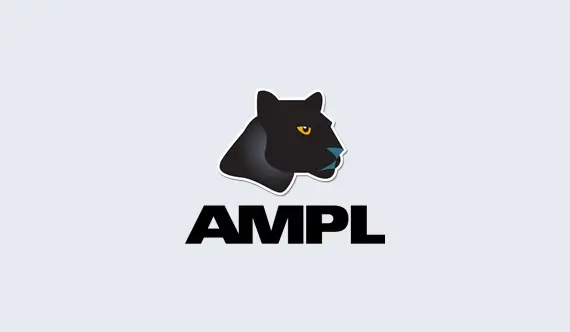
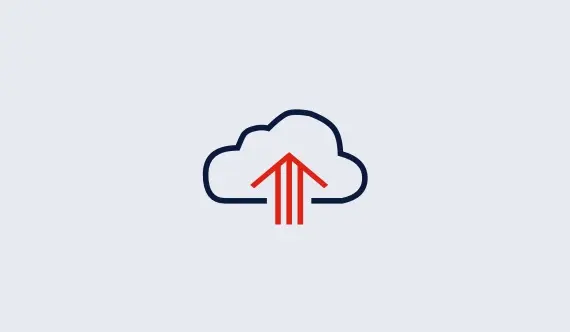
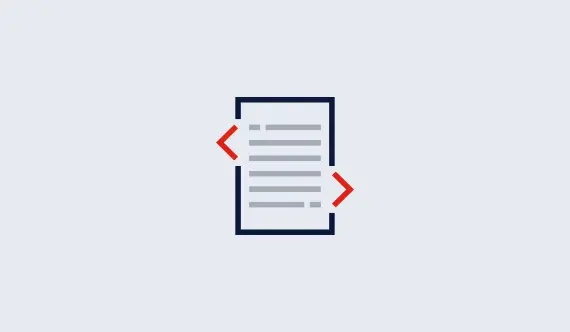
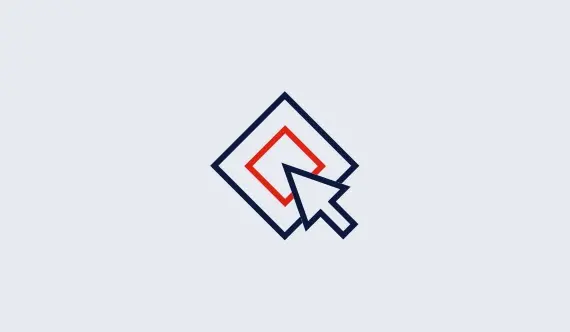
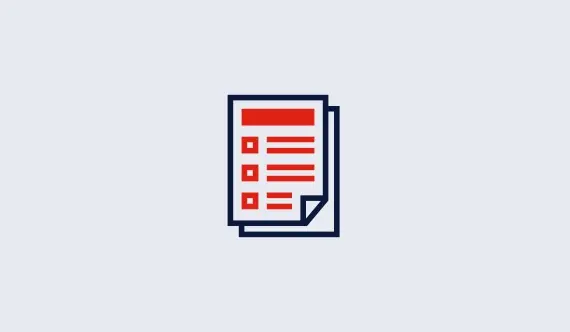
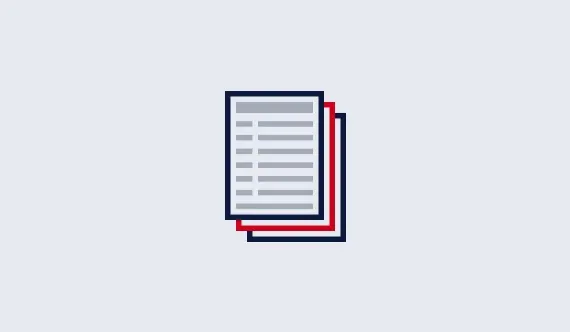
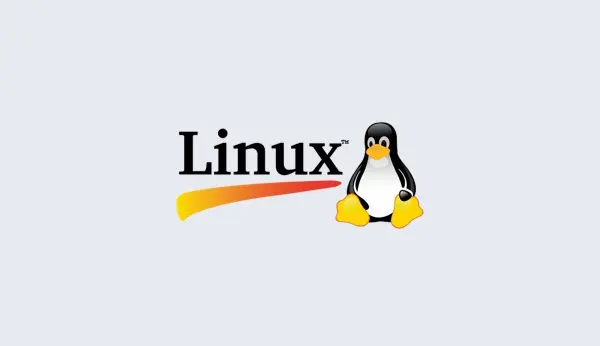
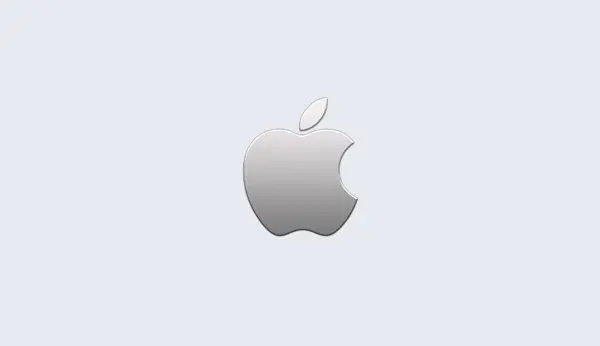
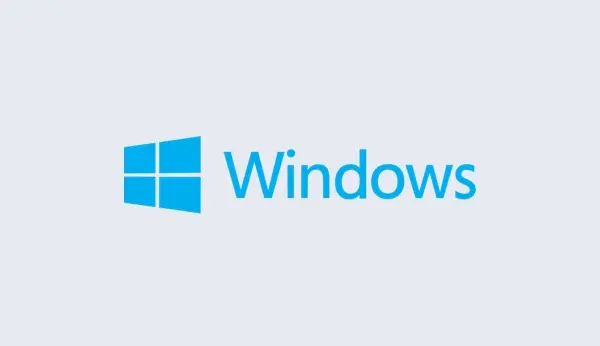