Dictionaries
A Python dictionary
allows you to map arbitrary key
values to pieces of data. Any immutable Python object can be
used as a key: an integer, a floating-point number, a string, or even
a tuple.
To give an example, the following statements create a dictionary x
,
and then associates a value 1
with key
('Pens', 'Denver', 'New York')
gurobi> x = {} # creates an empty dictionary gurobi> x[('Pens', 'Denver', 'New York')] = 1 gurobi> print x[('Pens', 'Denver', 'New York')] 1Python allows you to omit the parenthesis when accessing a dictionary using a tuple, so the following is also valid:
gurobi> x = {} gurobi> x['Pens', 'Denver', 'New York'] = 2 gurobi> print x['Pens', 'Denver', 'New York'] 2We've stored integers in the dictionary here, but dictionaries can hold arbitrary objects. In particular, they can hold Gurobi decision variables:
gurobi> x['Pens', 'Denver', 'New York'] = model.addVar() gurobi> print x['Pens', 'Denver', 'New York'] <gurobi.Var *Awaiting Model Update*>
To initialize a dictionary, you can of course simply perform assignments for each relevant key:
gurobi> values = {} gurobi> values['zero'] = 0 gurobi> values['one'] = 1 gurobi> values['two'] = 2You can also use the Python dictionary initialization construct:
gurobi> values = { 'zero': 0, 'one': 1, 'two': 2 } gurobi> print values['zero'] 0 gurobi> print values['one'] 1
We have included a utility routine in the Gurobi Python interface that
simplifies dictionary initialization for a case that arises frequently
in mathematical modeling. The multidict
function allows you
to initialize one or more dictionaries in a single statement. The
function takes a dictionary as its argument, where the value
associated with each key is a list of length n
. The function
splits these lists into individual entries, creating n
separate dictionaries. The function returns a list. The first
result is the list of shared key values, followed by the n
individual dictionaries:
gurobi> names, lower, upper = multidict({ 'x': [0, 1], 'y': [1, 2], 'z': [0, 3] }) gurobi> print names ['x', 'y', 'z'] gurobi> print lower {'x': 0, 'y': 1, 'z': 0} gurobi> print upper {'x': 1, 'y': 2, 'z': 3}Note that you can also apply this function to a dictionary where each key maps to a scalar value. In that case, the function simply returns the list of keys as the first result, and the original dictionary as the second.
You will see this function in several of our Python examples.
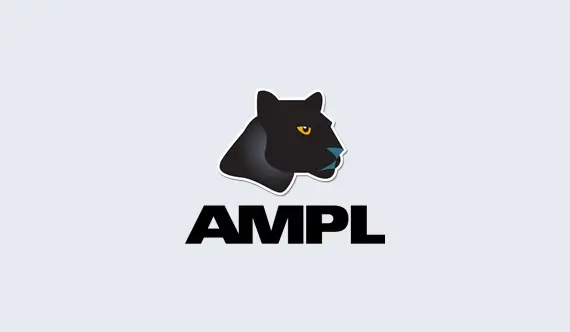
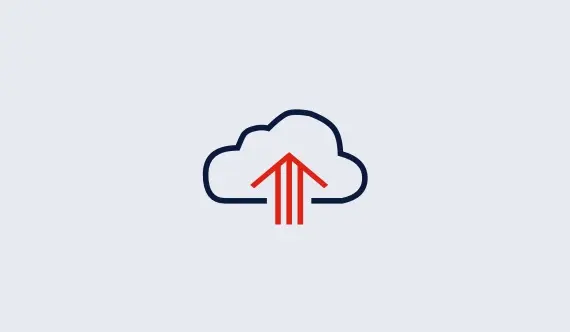
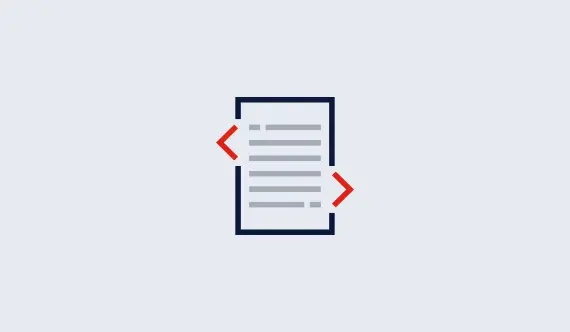
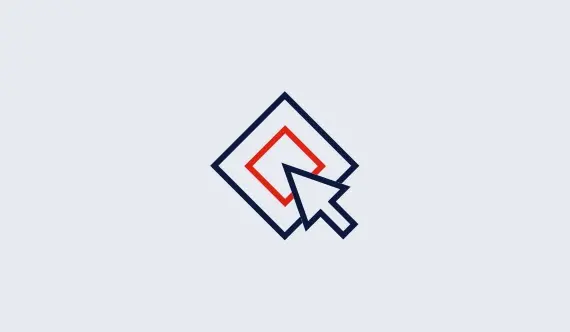
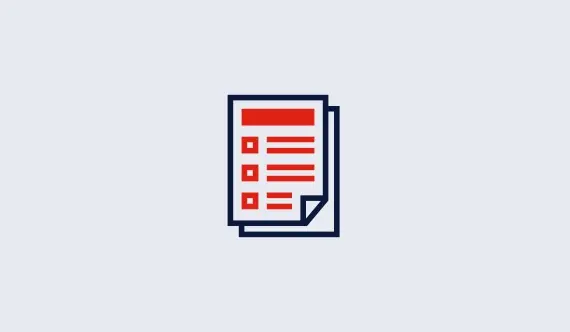
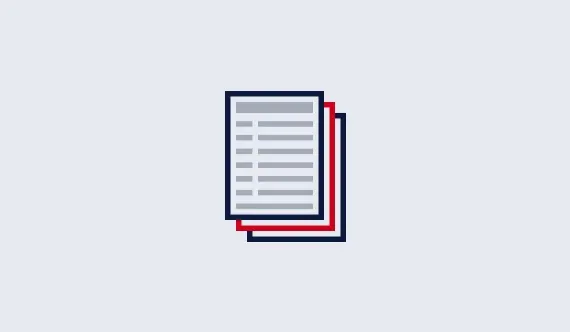
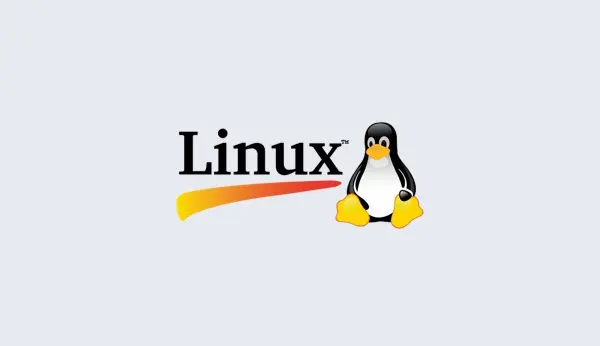
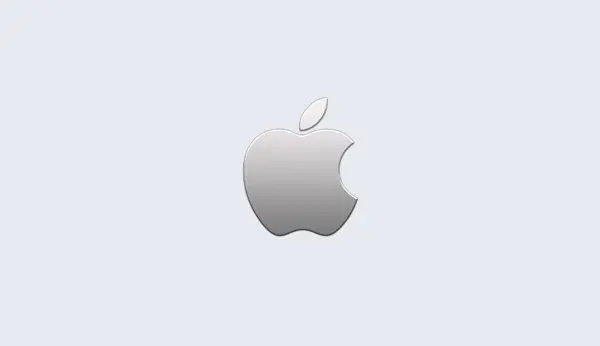
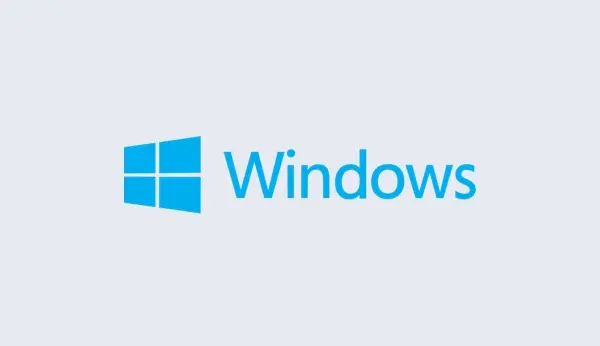