The tuplelist class
The final important item we would like to discuss is the
tuplelist
class. This is a custom sub-class of the Python
list
class that is designed to allow
you to efficiently build sub-lists from a list of tuples.
To be more specific, you can
use the select
method on a tuplelist
object to
retrieve all tuples that match one or more specified values
in specified fields.
Let us give a simple example. We'll begin by creating a simple
tuplelist
(by passing a list of tuples to the constructor):
gurobi> l = tuplelist([(1, 2), (1, 3), (2, 3), (2, 4)])To select a sub-list where particular tuple entries match desired values, you specify the desired values as arguments to the
select
method.
The number of arguments to select
is equal
to the number of entries in the members of the tuplelist
(they should all have the same number of entries).
You use a
'*'
string to indicate that any value is acceptable in that position
in the tuple.
Each tuple in our example contains two entries, so we can perform the following selections:
gurobi> print l.select(1, '*') [(1, 2), (1, 3)] gurobi> print l.select('*', 3) [(1, 3), (2, 3)] gurobi> print l.select(1, 3) [(1, 3)] gurobi> print l.select('*', '*') [(1, 2), (1, 3), (2, 3), (2, 4)]
You may have noticed that similar results could have been achieved using list comprehension. For example:
gurobi> print l.select(1, '*') [(1, 2), (1, 3)] gurobi> print [(x,y) for x,y in l if x == 1] [(1, 2), (1, 3)]The problem is that the latter statement considers every member in the list, which can be quite inefficient for large lists. The
select
method builds internal data structures that make these
selections quite efficient.
Note that tuplelist
is a sub-class of list
, so you can
use the standard list
methods to access or modify a
tuplelist
:
gurobi> print l[1] (1,3) gurobi> l += [(3, 4)] gurobi> print l [(1, 2), (1, 3), (2, 3), (2, 4), (3, 4)]
Returning to our network flow example, once we've built a
tuplelist
containing all valid commodity-source-destination
combinations on the network (we'll call it flows
), we
can select
all arcs that flow into a specific destination city as follows:
gurobi> inbound = flows.select('*', '*', 'New York')
We now present an example that illustrates the use of all of the concepts discussed so far.
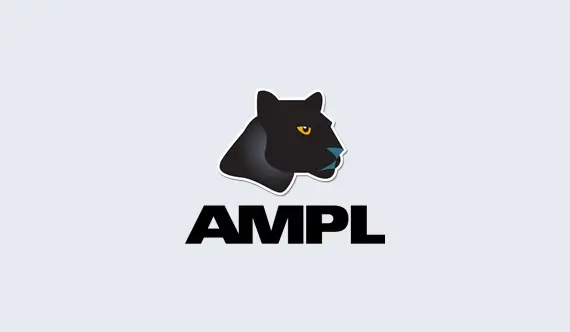
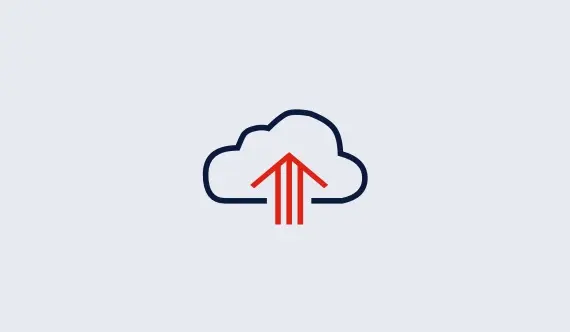
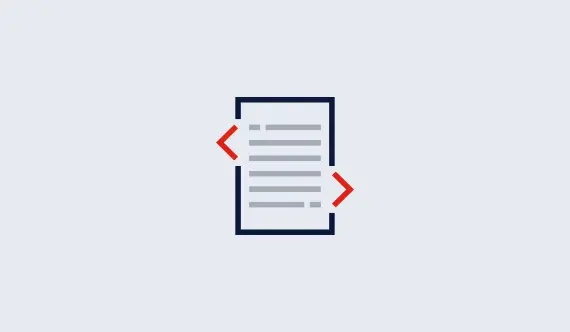
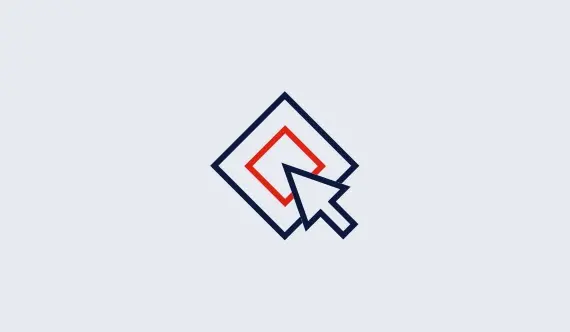
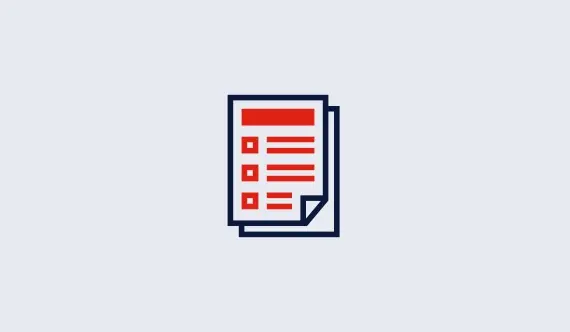
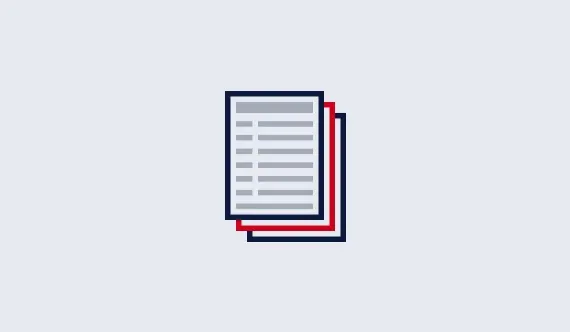
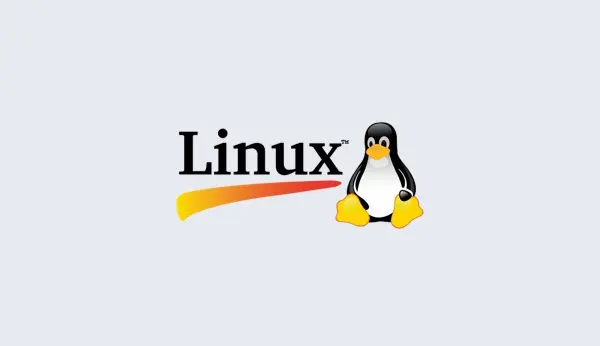
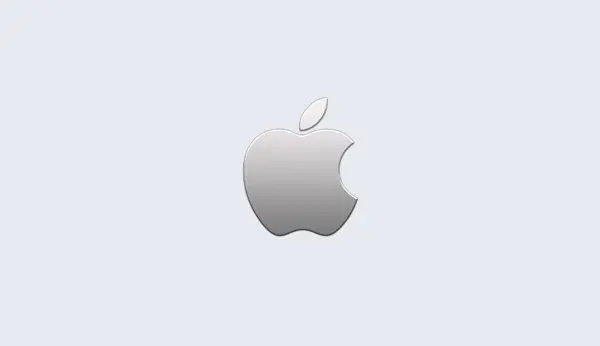
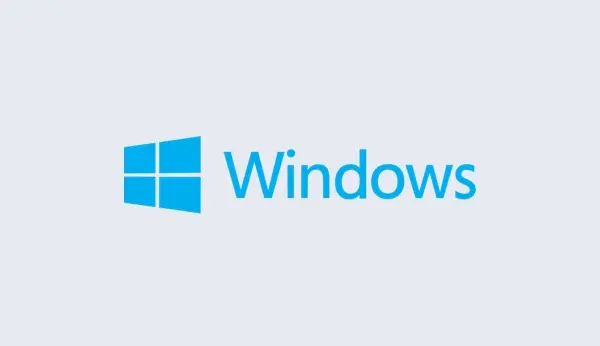