Filter Content By
Version
Text Search
${sidebar_list_label} - Back
Filter by Language
Constants
The following list contains the set of constants needed by the Gurobi
.NET interface. You would refer to them using a GRB.
prefix
(e.g., GRB.Status.OPTIMAL
).
// Model status codes (after call to optimize()) /// <summary> /// Optimization status codes /// </summary> public class Status { /// <summary> /// Model is loaded, but no solution information is available. /// </summary> public const int LOADED = 1; /// <summary> /// Model was solved to optimality (subject to tolerances) and an optimal /// solution is available. /// </summary> public const int OPTIMAL = 2; /// <summary> /// Model was proven to be infeasible. /// </summary> public const int INFEASIBLE = 3; /// <summary> /// Model was proven to be either infeasible or unbounded. /// </summary> public const int INF_OR_UNBD = 4; /// <summary> /// Model was proven to be unbounded. /// </summary> public const int UNBOUNDED = 5; /// <summary> /// Optimal objective was proven to be worse than the value specified /// in the Cutoff parameter. /// </summary> public const int CUTOFF = 6; /// <summary> /// Optimization terminated because the total number of simplex /// iterations performed exceeded the limit specified in the IterationLimit /// parameter. /// </summary> public const int ITERATION_LIMIT = 7; /// <summary> /// Optimization terminated because the total number of branch-and-cut /// nodes explored exceeded the limit specified in the NodeLimit /// parameter. /// </summary> public const int NODE_LIMIT = 8; /// <summary> /// Optimization terminated because the total elapsed time /// exceeded the limit specified in the TimeLimit parameter. /// </summary> public const int TIME_LIMIT = 9; /// <summary> /// Optimization terminated because the number of solutions found /// reached the value specified in the SolutionLimit parameter. /// </summary> public const int SOLUTION_LIMIT = 10; /// <summary> /// Optimization was terminated by the user. /// </summary> public const int INTERRUPTED = 11; /// <summary> /// Optimization was terminated due to unrecoverable numerical /// difficulties. /// </summary> public const int NUMERIC = 12; /// <summary> /// Optimization terminated with a sub-optimal solution. /// </summary> public const int SUBOPTIMAL = 13; /// <summary> /// Optimization is still in progress. /// </summary> public const int INPROGRESS = 14; /// <summary> /// User specified objective limit (a bound on either the best objective /// or the best bound), and that limit has been reached. /// </summary> public const int USER_OBJ_LIMIT = 15; } /// <summary> /// Batch status codes /// </summary> public class BatchStatus { /// <summary> /// Batch object was created, but is not ready to be scheduled. /// See the Batch Optimization section of the manual for more details. /// </summary> public const int CREATED = 1; /// <summary> /// The Batch object has been completely specified, and now is waiting /// for a job to finish processing the request. /// See the Batch Optimization section of the manual for more details. /// </summary> public const int SUBMITTED = 2; /// <summary> /// Batch processing was aborted by the user. /// See the Batch Optimization section of the manual for more details. /// </summary> public const int ABORTED = 3; /// <summary> /// Batch processing failed. /// See the Batch Optimization section of the manual for more details. /// </summary> public const int FAILED = 4; /// <summary> /// A Batch Job successfully processed the Batch request. /// See the Batch Optimization section of the manual for more details. /// </summary> public const int COMPLETED = 5; } // Version numbers /// <summary> /// Major number /// </summary> public const int VERSION_MAJOR = 9; /// <summary> /// Minor number /// </summary> public const int VERSION_MINOR = 1; /// <summary> /// Technical number /// </summary> public const int VERSION_TECHNICAL = 2; // Basis status info /// <summary> /// Variable is basic. /// </summary> public const int BASIC = 0; /// <summary> /// Variable is non-basic at lower bound. /// </summary> public const int NONBASIC_LOWER = -1; /// <summary> /// Variable is non-basic at upper bound. /// </summary> public const int NONBASIC_UPPER = -2; /// <summary> /// Variable is superbasic. /// </summary> public const int SUPERBASIC = -3; // Constraint senses public const char LESS_EQUAL = '<'; public const char GREATER_EQUAL = '>'; public const char EQUAL = '='; // Variable types public const char CONTINUOUS = 'C'; public const char BINARY = 'B'; public const char INTEGER = 'I'; public const char SEMICONT = 'S'; public const char SEMIINT = 'N'; // Objective sense public const int MINIMIZE = 1; public const int MAXIMIZE = -1; // SOS types public const int SOS_TYPE1 = 1; public const int SOS_TYPE2 = 2; // General constraint types public const int GENCONSTR_MAX = 0; public const int GENCONSTR_MIN = 1; public const int GENCONSTR_ABS = 2; public const int GENCONSTR_AND = 3; public const int GENCONSTR_OR = 4; public const int GENCONSTR_INDICATOR = 5; public const int GENCONSTR_PWL = 6; public const int GENCONSTR_POLY = 7; public const int GENCONSTR_EXP = 8; public const int GENCONSTR_EXPA = 9; public const int GENCONSTR_LOG = 10; public const int GENCONSTR_LOGA = 11; public const int GENCONSTR_POW = 12; public const int GENCONSTR_SIN = 13; public const int GENCONSTR_COS = 14; public const int GENCONSTR_TAN = 15; // Numeric constants public const double INFINITY = 1e100; public const double UNDEFINED = 1e101; public const int MAXINT = 2000000000; // Other constants public const int DEFAULT_CS_PORT = 61000; // Limits public const int MAX_STRLEN = 512; public const int MAX_NAMELEN = 255; public const int MAX_TAGLEN = 10240; public const int MAX_CONCURRENT = 64; // Callback constants /// <summary> /// Gurobi callback codes. /// </summary> public class Callback { /// <summary> /// Periodic polling callback. /// </summary> public const int POLLING = 0; /// <summary> /// Currently performing presolve. /// </summary> public const int PRESOLVE = 1; /// <summary> /// Currently in simplex. /// </summary> public const int SIMPLEX = 2; /// <summary> /// Currently in MIP. /// </summary> public const int MIP = 3; /// <summary> /// Found a new MIP incumbent. /// </summary> public const int MIPSOL = 4; /// <summary> /// Currently exploring a MIP node. /// </summary> public const int MIPNODE = 5; /// <summary> /// Currently in barrier. /// </summary> public const int BARRIER = 7; /// <summary> /// Printing a log message. /// </summary> public const int MESSAGE = 6; /// <summary> /// Currently in multi-objective optimization. /// </summary> public const int MULTIOBJ = 8; /// <summary> /// Returns the number of columns removed by presolve to this point. /// </summary> public const int PRE_COLDEL = 1000; /// <summary> /// Returns the number of rows removed by presolve to this point. /// </summary> public const int PRE_ROWDEL = 1001; /// <summary> /// Returns the number of constraint senses changed by presolve to this /// point. /// </summary> public const int PRE_SENCHG = 1002; /// <summary> /// Returns the number of bounds changed by presolve to this point. /// </summary> public const int PRE_BNDCHG = 1003; /// <summary> /// Returns the number of coefficients changed by presolve to this point. /// </summary> public const int PRE_COECHG = 1004; /// <summary> /// Returns the current simplex iteration count. /// </summary> public const int SPX_ITRCNT = 2000; /// <summary> /// Returns the current simplex objective value. /// </summary> public const int SPX_OBJVAL = 2001; /// <summary> /// Returns the current simplex primal infeasibility. /// </summary> public const int SPX_PRIMINF = 2002; /// <summary> /// Returns the current simplex dual infeasibility. /// </summary> public const int SPX_DUALINF = 2003; /// <summary> /// Returns 1 if the model has been perturbed. /// </summary> public const int SPX_ISPERT = 2004; /// <summary> /// Returns the current best objective value. /// </summary> public const int MIP_OBJBST = 3000; /// <summary> /// Returns the current best objective bound. /// </summary> public const int MIP_OBJBND = 3001; /// <summary> /// Returns the current explored node count. /// </summary> public const int MIP_NODCNT = 3002; /// <summary> /// Returns the current solution count. /// </summary> public const int MIP_SOLCNT = 3003; /// <summary> /// Returns the current cutting plane count. /// </summary> public const int MIP_CUTCNT = 3004; /// <summary> /// Returns the current unexplored node count. /// </summary> public const int MIP_NODLFT = 3005; /// <summary> /// Returns the current simplex iteration count. /// </summary> public const int MIP_ITRCNT = 3006; /// <summary> /// Returns the new solution. /// </summary> public const int MIPSOL_SOL = 4001; /// <summary> /// Returns the objective value for the new solution. /// </summary> public const int MIPSOL_OBJ = 4002; /// <summary> /// Returns the current best objective value. /// </summary> public const int MIPSOL_OBJBST = 4003; /// <summary> /// Returns the current best objective bound. /// </summary> public const int MIPSOL_OBJBND = 4004; /// <summary> /// Returns the current explored node count. /// </summary> public const int MIPSOL_NODCNT = 4005; /// <summary> /// Returns the current solution count. /// </summary> public const int MIPSOL_SOLCNT = 4006; /// <summary> /// Returns the status of the current node relaxation. /// </summary> public const int MIPNODE_STATUS = 5001; /// <summary> /// Returns the current node relaxation solution or ray. /// </summary> public const int MIPNODE_REL = 5002; /// <summary> /// Returns the current best objective value. /// </summary> public const int MIPNODE_OBJBST = 5003; /// <summary> /// Returns the current best objective bound. /// </summary> public const int MIPNODE_OBJBND = 5004; /// <summary> /// Returns the current explored node count. /// </summary> public const int MIPNODE_NODCNT = 5005; /// <summary> /// Returns the current solution count. /// </summary> public const int MIPNODE_SOLCNT = 5006; /// <summary> /// Returns the branching variable for the current node. /// </summary> public const int MIPNODE_BRVAR = 5007; /// <summary> /// Returns the current barrier iteration count. /// </summary> public const int BARRIER_ITRCNT = 7001; /// <summary> /// Returns the current barrier primal objective value. /// </summary> public const int BARRIER_PRIMOBJ = 7002; /// <summary> /// Returns the current barrier dual objective value. /// </summary> public const int BARRIER_DUALOBJ = 7003; /// <summary> /// Returns the current barrier primal infeasibility. /// </summary> public const int BARRIER_PRIMINF = 7004; /// <summary> /// Returns the current barrier dual infeasibility. /// </summary> public const int BARRIER_DUALINF = 7005; /// <summary> /// Returns the current barrier complementarity violation. /// </summary> public const int BARRIER_COMPL = 7006; /// <summary> /// Returns the message that is being printed. /// </summary> public const int MSG_STRING = 6001; /// <summary> /// Returns the elapsed solver runtime (in seconds). /// </summary> public const int RUNTIME = 6002; /// <summary> /// Returns the current objective count already optimized. /// </summary> public const int MULTIOBJ_OBJCNT = 8001; /// <summary> /// Returns the current solution count. /// </summary> public const int MULTIOBJ_SOLCNT = 8002; /// <summary> /// Returns the new solution. /// </summary> public const int MULTIOBJ_SOL = 8003; } // Errors /// <summary> /// Gurobi error codes. /// </summary> public class Error { /// <summary> /// Available memory was exhausted. /// </summary> public const int OUT_OF_MEMORY = 10001; /// <summary> /// NULL input value provided for a required argument. /// </summary> public const int NULL_ARGUMENT = 10002; /// <summary> /// Invalid input value. /// </summary> public const int INVALID_ARGUMENT = 10003; /// <summary> /// Tried to query or set an unknown attribute. /// </summary> public const int UNKNOWN_ATTRIBUTE = 10004; /// <summary> /// Tried to query or set an attribute that could not be accessed. /// </summary> public const int DATA_NOT_AVAILABLE = 10005; /// <summary> /// Index for attribute query was out of range. /// </summary> public const int INDEX_OUT_OF_RANGE = 10006; /// <summary> /// Tried to query or set an unknown parameter. /// </summary> public const int UNKNOWN_PARAMETER = 10007; /// <summary> /// Tried to set a parameter to a value that is outside its valid range. /// </summary> public const int VALUE_OUT_OF_RANGE = 10008; /// <summary> /// Failed to obtain a Gurobi license. /// </summary> public const int NO_LICENSE = 10009; /// <summary> /// Attempted to solve a model that is larger than the limit for a /// trial license. /// </summary> public const int SIZE_LIMIT_EXCEEDED = 10010; /// <summary> /// Problem in callback. /// </summary> public const int CALLBACK = 10011; /// <summary> /// Failed to read the requested file. /// </summary> public const int FILE_READ = 10012; /// <summary> /// Failed to write the requested file. /// </summary> public const int FILE_WRITE = 10013; /// <summary> /// Numerical error during requested operation. /// </summary> public const int NUMERIC = 10014; /// <summary> /// Attempted to perform infeasibility analysis on a feasible model. /// </summary> public const int IIS_NOT_INFEASIBLE = 10015; /// <summary> /// Requested operation not valid for a MIP model. /// </summary> public const int NOT_FOR_MIP = 10016; /// <summary> /// Tried to access a model while optimization was in progress. /// </summary> public const int OPTIMIZATION_IN_PROGRESS = 10017; /// <summary> /// Constraint, variable, or SOS contained duplicate indices. /// </summary> public const int DUPLICATES = 10018; /// <summary> /// Error in reading or writing a MIP node file. /// </summary> public const int NODEFILE = 10019; /// <summary> /// non-PSD Q matrix in the objective or in a quadratic constraint. /// </summary> public const int Q_NOT_PSD = 10020; /// <summary> /// Equality quadratic constraints. /// </summary> public const int QCP_EQUALITY_CONSTRAINT = 10021; /// <summary> /// Network error. /// </summary> public const int NETWORK = 10022; /// <summary> /// Job rejected from Compute Server queue. /// </summary> public const int JOB_REJECTED = 10023; /// <summary> /// Operation is not supported in the current usage environment. /// </summary> public const int NOT_SUPPORTED = 10024; /// <summary> /// Result is larger than return value allows. /// </summary> public const int EXCEED_2B_NONZEROS = 10025; /// <summary> /// Problem with piecewise-linear objective function. /// </summary> public const int INVALID_PIECEWIE_OBJ = 10026; /// <summary> /// Not allowed to change UpdateMode parameter once model /// has been created. /// </summary> public const int UPDATEMODE_CHANGE = 10027; /// <summary> /// Problem launching Instant Cloud job. /// </summary> public const int CLOUD = 10028; /// <summary> /// An error occurred during model modification or update. /// </summary> public const int MODEL_MODIFICATION = 10029; /// <summary> /// An error occured with the client-server application. /// </summary> public const int CSWORKER = 10030; /// <summary> /// Multi-model tuning invoked on models of different types. /// </summary> public const int TUNE_MODEL_TYPES = 10031; /// <summary> /// Multi-model tuning invoked on models of different types. /// </summary> public const int SECURITY = 10032; /// <summary> /// Tried to access a constraint or variable that is not in the model. /// </summary> public const int NOT_IN_MODEL = 20001; /// <summary> /// Failed to create the requested model. /// </summary> public const int FAILED_TO_CREATE_MODEL = 20002; /// <summary> /// Internal Gurobi error. /// </summary> public const int INTERNAL = 20003; } /// <summary> /// Constant for Method parameter - /// choose method automatically. /// </summary> public const int METHOD_AUTO = -1; /// <summary> /// Constant for Method and NodeMethod parameters - /// use primal simplex. /// </summary> public const int METHOD_PRIMAL = 0; /// <summary> /// Constant for Method and NodeMethod parameters - /// use dual simplex. /// </summary> public const int METHOD_DUAL = 1; /// <summary> /// Constant for Method and NodeMethod parameters - /// use barrier. /// </summary> public const int METHOD_BARRIER = 2; /// <summary> /// Constant for Method parameters - use concurrent optimizer. /// </summary> public const int METHOD_CONCURRENT = 3; /// <summary> /// Constant for Method parameter - use deterministic concurrent optimizer. /// </summary> public const int METHOD_DETERMINISTIC_CONCURRENT = 4; /// <summary> /// minimize linearly weighted sum of penalties for feasrelax model. /// </summary> public const int FEASRELAX_LINEAR = 0; /// <summary> /// minimize quadratically weighted sum of penalties for feasrelax model. /// </summary> public const int FEASRELAX_QUADRATIC = 1; /// <summary> /// minimize weighted cardinality of relaxations for feasrelax model. /// </summary> public const int FEASRELAX_CARDINALITY = 2;
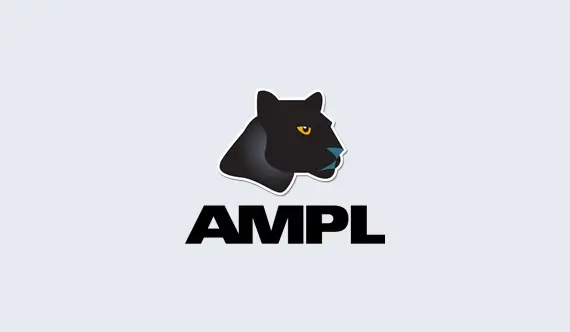
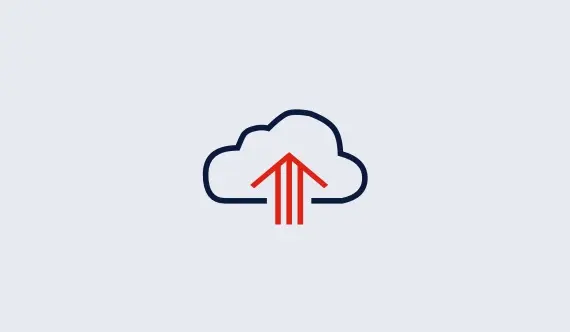
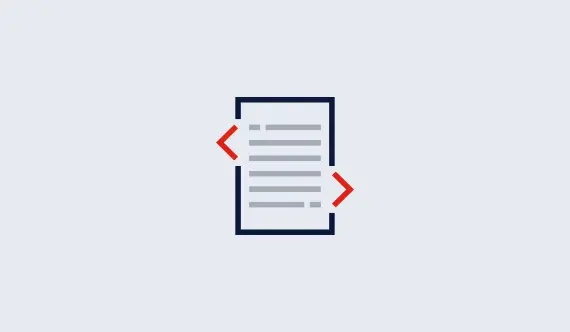
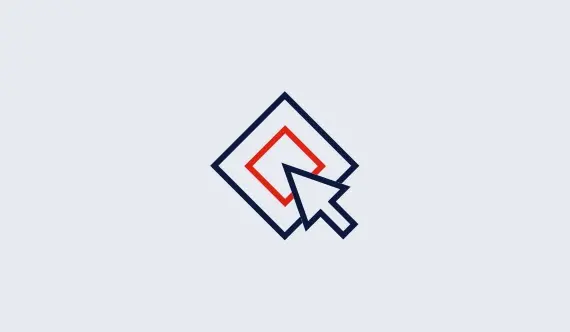
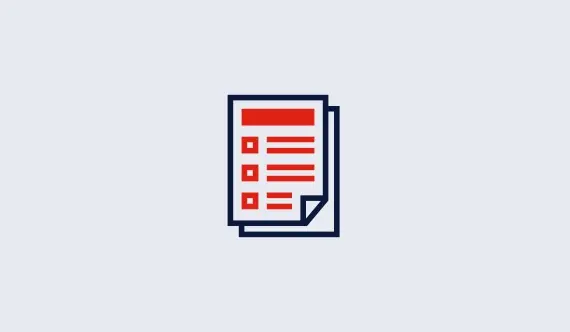
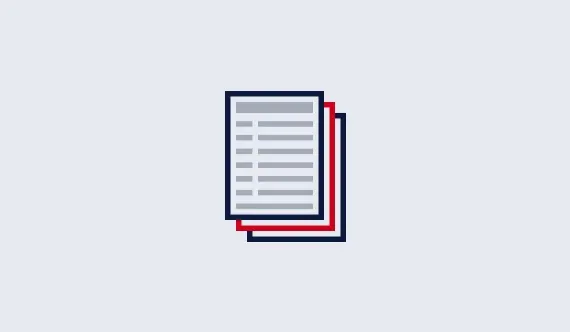
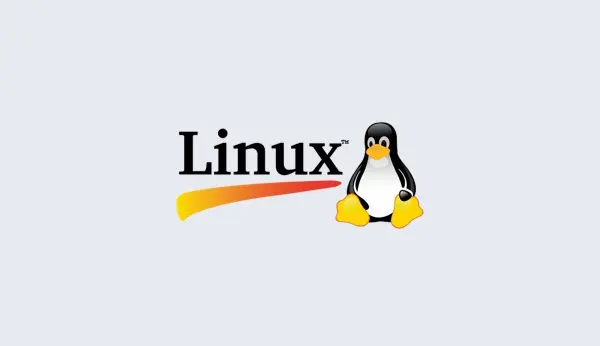
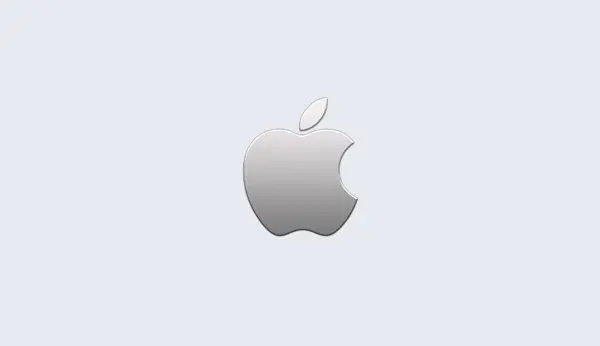
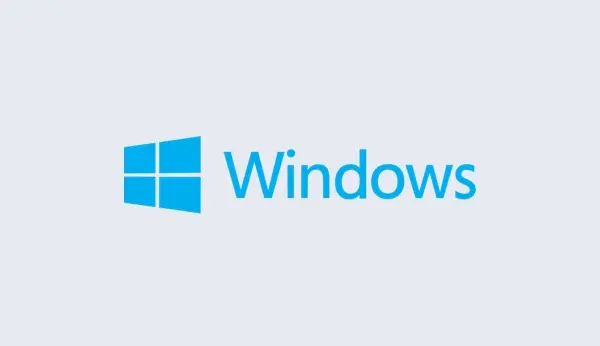